This is the second part of the series where I describe automating the scheduling of each Sunday’s live streams on my church’s YouTube channel.
- Part 1 – Parsing the Canadian Catholic liturgical calendar
- Part 2 – Creating YouTube live streams programmatically
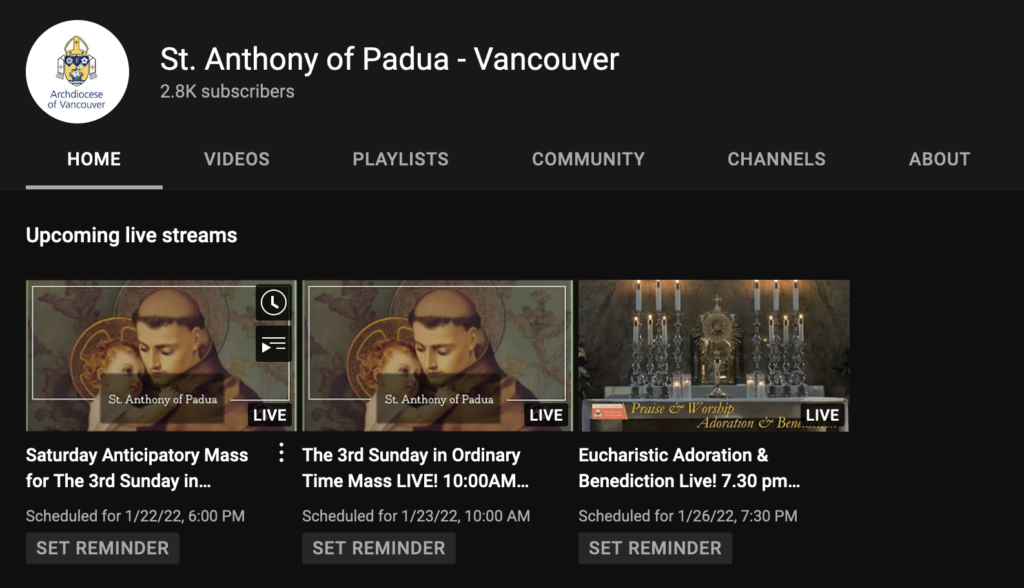
Part 2 – Creating YouTube live streams programmatically
Authentication with the YouTube Data API from Python
This is the second project for which I’ve had to go through and figure this out, so I wanted to write this down. There are a couple oddities with the YouTube Data API:
- Normally, server-to-server API calls to Google APIs without any user interface use “service accounts”. However, for the YouTube Data API, OAuth 2.0 has to be used instead of service accounts.
- The YouTube Live Stream API exists through the YouTube Data API.
The first part generally follows the Python Quickstart guide.
- First, install the following Python packages:
pip install google-api-python-client google-auth google-auth-oauthlib
- Second, on the Google API Console:
- Create or select a project.
- Enable the YouTube Data API v3 from the API Library.
- On the Credentials panel, create an “OAuth client ID”.
- It may ask you to “Configure Consent Screen” first – continue with that if asked:
- The “User Type” can be External.
- The next page will ask what you want the consent screen to look like – just fill out the essentials as this will only be seen by you to generate the initial token.
- On the Scopes page, add the following scopes:
https://www.googleapis.com/auth/youtube
https://www.googleapis.com/auth/youtube.force-ssl
https://www.googleapis.com/auth/youtube.upload
- On the test user page, you’ll want to add your own account, or the account that you want to create the YouTube videos in. This is important so that the OAuth login will work without being approved by Google.
- Continuing with the Credential creation, for the Application Type, select “Desktop app”. The client ID and client secret will now be displayed. Download the JSON version (which we’ll call
client_secrets.json
) of this which will be loaded in the code.
- Click on OAuth consent screen on the left bar. Under “Publishing status”, click on “Publish App”. This is the key in order to allow refresh tokens to be valid for more than a week.
The rest of our authentication can be invoked through code. Using the client_secrets.json
, we will use that to go through the OAuth 2.0 login flow to save a refresh token. This refresh token will then be used from time to time to exchange for an access token, which is a short-lived token used to call the APIs during one session.
from google_auth_oauthlib.flow import InstalledAppFlow def generate_authorized_user_file(): """ Run the authentication flow and save the token (refresh token) for future refreshes """ flow = InstalledAppFlow.from_client_secrets_file( "client_secrets.json", scopes=[ "https://www.googleapis.com/auth/youtube", "https://www.googleapis.com/auth/youtube.force-ssl", "https://www.googleapis.com/auth/youtube.upload", ], ) credentials = flow.run_console() with open("token.json", "w", encoding="UTF-8") as token_file: token_file.write(credentials.to_json()) return credentials
You’ll need to run this function in an interactive shell, because it will prompt you with a URL to complete the login process.
The browser portion looks something like this. After logging into your account, Google will say that it hasn’t verified this app. This is fine because we just created this project above, and we don’t expect to show this to the public, so just click “Continue”.
On the next screen, select all the permissions and then click “Continue”.
Finally, there will be a code you will copy back into the command line console.
Once you copy it back, the function will write the details of the refresh token into a token.js
file. The above process should only need to be done once initially. The resulting token can now be used in the following function, which will be called each time an API needs to be called (the following parts don’t need browser/console interactivity).
import google from google.oauth2.credentials import Credentials def refresh_credentials(): """ Returns current credentials from saved refresh token """ credentials = Credentials.from_authorized_user_file("token.json") credentials.refresh(google.auth.transport.requests.Request()) return credentials
Calling the YouTube APIs
Now with the credentials, the YouTube APIs can be called. Here is an example for scheduling a live stream. The documentation for the liveBroadcasts.insert
API can be found here.
from googleapiclient.discovery import build livestream_details = { "snippet": { "title": "Stream title", "scheduledStartTime": "2022-02-01T16:00+00Z", }, "status": { "privacyStatus": "public", }, } api = build("youtube", "v3", credentials=refresh_credentials()) api.liveBroadcasts().insert( part="snippet,status", body=livestream_details, ).execute()
Conclusion
I hope that gives some concrete starting points for writing Python scripts to interact with YouTube’s APIs, and in particular the live streaming APIs.
Congrats, Dennis, for this fantastic post. It is just what I needed.
I have developed a python script in order to create a live stream programatically. It performs good, everyday my script creates a new live stream for streaming on youtube the Holy Mass and Liturgy.
But after a week the refresh token expires… I wanted to publish my app but I don’t know how should I proceed.
A windows appears asking for:
– An official link to your app’s Privacy Policy
– A YouTube video showing how you plan to use the Google user data you get from scopes
– A written explanation telling Google why you need access to sensitive and/or restricted user data
– All your domains verified in Google Search Console
This is only a python script. It has no GUI nor link, it is a Desktop App…
Please, Dennis, have you managed to get published your App? What information have you delivered to Youtube in order to get your app verified and published?
I wil appreciate a lot your help. Thanks, Dennis
Hi Julio,
Yes I encountered this issue as well. The key was to publish the OAuth Consent Screen. However, it is not necessary to go through the verification process. That is, it just needs to be published (not in testing mode) and unverified.
Hope that helps. God bless.